Loading and Accepting Friend Requests
1. Load and accept friend requests sent to me
Add content to the GetReceivedRequestFriend and ApplyFriend methods in BackendFriend.cs written in the Pre-arrangements stage.
BackendFriend.cs
Before editing
public void GetReceivedRequestFriend()
{
// Adds logic of Step 3. Loading and Accepting Friend Requests (part on loading)
}
public void ApplyFriend(int index)
{
// Adds logic of Step 3. Loading and Accepting Friend Requests (part on accepting)
}
After editing
public void GetReceivedRequestFriend()
{
var bro = Backend.Friend.GetReceivedRequestList();
if (bro.IsSuccess() == false)
{
Debug.Log("친구 요청 받은 리스트를 불러오는 중 에러가 발생했습니다. : " + bro);
return;
}
if (bro.FlattenRows().Count <= 0)
{
Debug.LogError("친구 요청이 온 내역이 존재하지 않습니다.");
return;
}
Debug.Log("친구 요청 받은 리스트 불러오기에 성공했습니다. : " + bro);
int index = 0;
foreach (LitJson.JsonData friendJson in bro.FlattenRows())
{
string nickName = friendJson["nickname"]?.ToString();
string inDate = friendJson["inDate"].ToString();
_requestFriendList.Add(new Tuple<string, string>(nickName, inDate));
Debug.Log($"{index}. {nickName} - {inDate}");
index++;
}
}
public void ApplyFriend(int index)
{
if (_requestFriendList.Count <= 0)
{
Debug.LogError("요청이 온 친구가 존재하지 않습니다.");
return;
}
if (index >= _requestFriendList.Count)
{
Debug.LogError($"요청한 친구 요청 리스트의 범위를 벗어났습니다. 선택 : {index} / 리스트 최대 : {_requestFriendList.Count}");
return;
}
var bro = Backend.Friend.AcceptFriend(_requestFriendList[index].Item2);
if (bro.IsSuccess() == false)
{
Debug.LogError("친구 수락 중 에러가 발생했습니다. : " + bro);
return;
}
Debug.Log($"{_requestFriendList[index].Item1}이(가) 친구가 되었습니다. : " + bro);
}
2. Add method call to BackendManager.cs
BackendManager, which is automatically called when the game is executed, must be used to call this method.\ Add it so that the method may be called after BACKND initialization and BACKND login.
로그인하는 유저는 Step2에서 로그인(회원가입)한 유저가 아닌 친구 요청을 보낸 유저로 로그인을 해야합니다.
BackendManager.cs
Before editing (Logic for Step 2. Sending Friend Requests)
void Test()
{
// user1에게 보낼 것이므로 user2로 회원가입
string user2Id = "user2";
// user2Id로 회원가입(409 에러 발생 시, 이미 user2Id로 아이디를 생성했으므로 CustomSignUp을 CustomLogin으로 변경)
BackendLogin.Instance.CustomSignUp(user2Id, "1234");
BackendLogin.Instance.UpdateNickname(user2Id); // 아이디와 동일하게 닉네임 변경
string user1Nickname = "원하는 이름"; // 유저1의 닉네임(유저에 따라 다를 수 있습니다.)
BackendFriend.Instance.SendFriendRequest(user1Nickname); // 친구 요청 보내기 함수
Debug.Log("테스트를 종료합니다.");
}
After editing
void Test()
{
// Must be logged in as the user to which user2 sent the friend request in Step 2.
BackendLogin.Instance.CustomLogin("user1", "1234");
BackendFriend.Instance.GetReceivedRequestFriend(); // Loads friend requests list
BackendFriend.Instance.ApplyFriend(0); // Accepts the latest friend request on the friend requests list
Debug.Log("Test complete.");
}
3. Test in Unity
After editing the script, execute Unity debugging and check the console log of Unity.
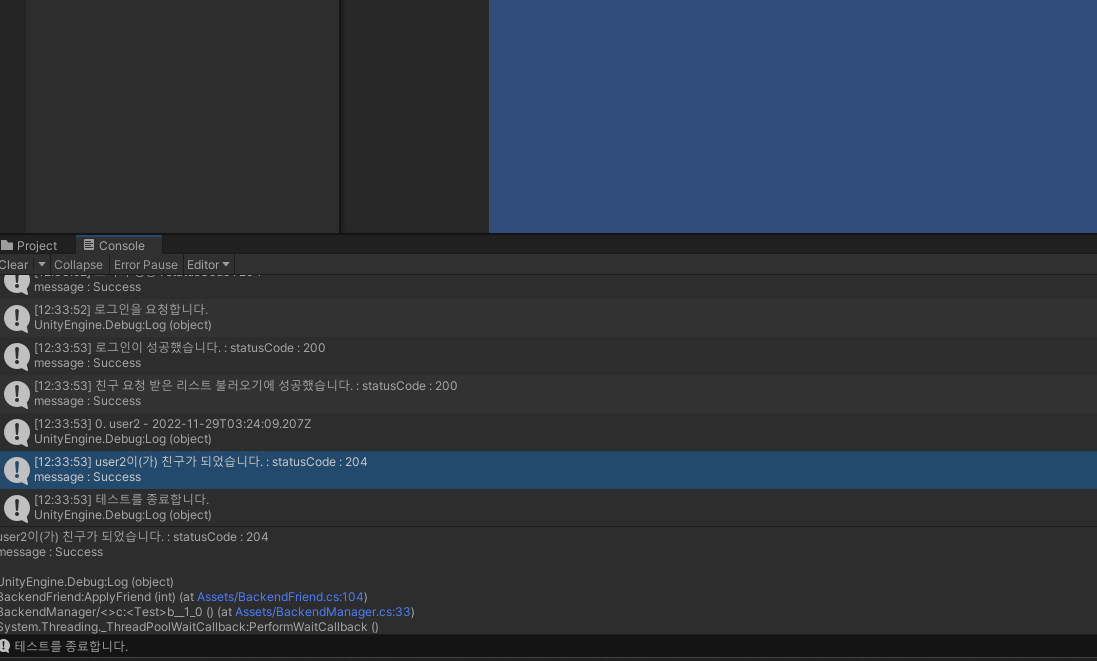
이때 로그에서 **'ㅇㅇ이(가) 친구가 되었습니다. : statusCode : 204'**이 표시되어야 함수 호출에 성공한 것입니다.\ When errors, such as statusCode : 400, 404, and 409, occur instead of the above log, you can check which errors caused the failure in GetReceivedRequestList error case and AcceptFriend error case.