Using Steamworks.NET
- Sign in to Steamworks to receive appID and Web API Key
- Add Steamworks.NET to Unity
- Enter the issued appID in steam_appid.txt which is in the Unity project folder
- Call the GetAuthSessionTicket method to receive a session ticket
- Use the issued session ticket to log in to the BACKND server
- This document was written based on the Release 20.0.0 version of Steamworks.NET.
- Since versions later than Release 20.0.0 have 1 more parameter for the GetAuthSessionTicket method, the example from the developer documentation cannot be used.
- If Steamworks.NET is added, unable to run on platforms other than Steam.
- If run on platforms other than Steam, automatically terminated and will be executed again through the Steam platform.
- For details, please refer to the Steamworks.NET developer documentation.
Initial settings
1. Register application to Steamworks
Access the Steamworks page and create an app. Then click Dashboard -> All Applications.
Click the Steamworks admin and go to Steamworks Admin > App Admin > (Project name), and then go to SteamPipe > Builds.
Click Here to upload the app. From Confirm Depot as Build, set the app as default and click Commit.
2. Issue appID
Go to Steamworks Admin > App Admin > (Project name). The number inside the parentheses refers to appID, which is used as a unique number for the app.
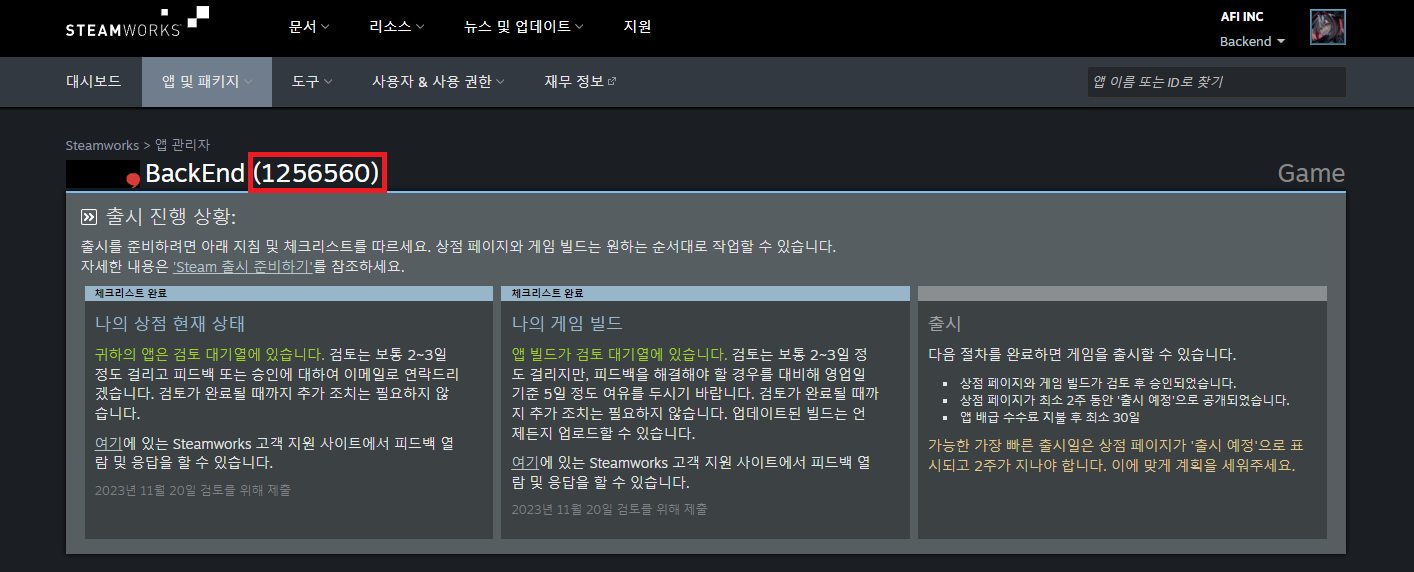
3. Issue Web API key and register app
You must first create a group to issue a Web API Key.
Go to Steamworks > Users & Permissions > Manage Groups, then click Create New Group.
Set the name of the group and click Create Group.
You will be moved to the group page if the group is successfully created. Click Create WebAPI Key to create a key.
Register an app to the group to use the created Web API key.
4. Register Steam account authentication information to the BACKND Console
Register the appID and Web API Key issued from the previous steps in BACKND Console > Authentication Information > Steam Account Authentication Information.
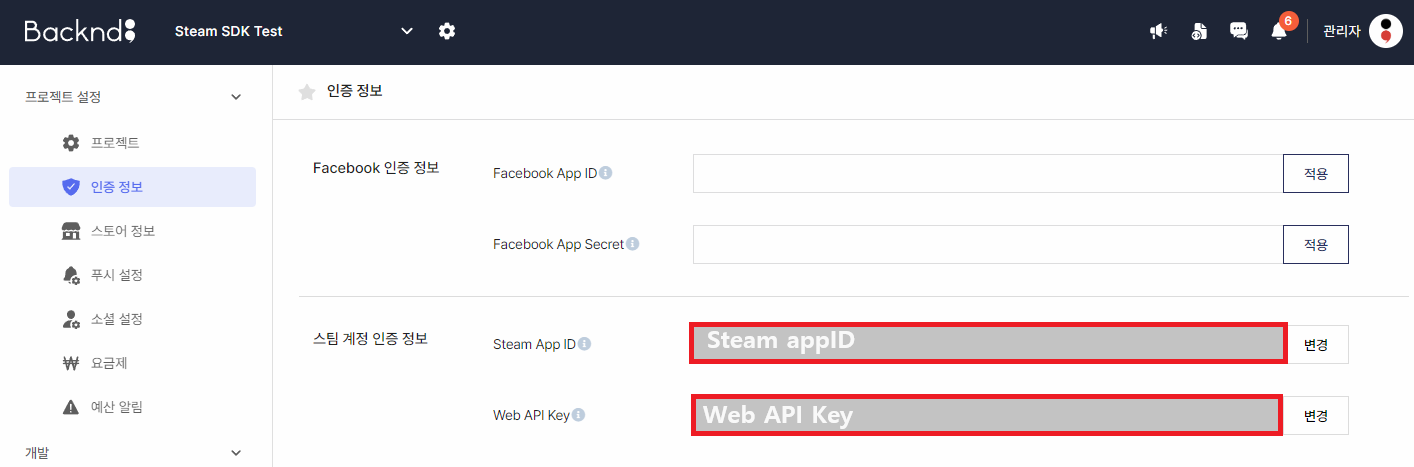
Invite users
To test an app before it is released, you need to invite users to the group and grant permissions.
1. Send user invitation mails and set permissions
Go to Steamworks > Users & Permissions > Manage Users, then click Invite User.
Set the email address to invite the user and configure the permissions of the user that will be invited. Then click Invite User.
If you have successfully completed the invitation process, an invitation mail will be sent to the entered email address.
If the user accepts the invitation, you can accept their request in Invitations Awaiting Confirmation. Click Accept to complete the invitation process.
2. Add to group
From Steamworks > Users & Permissions > Manage Groups, go to the group page created by following the previous steps.
Click Member List > Add User to add users to the group.
Unity settings
1. Install Steam SDK
Install Steamworks.NET and import it to the Unity project.
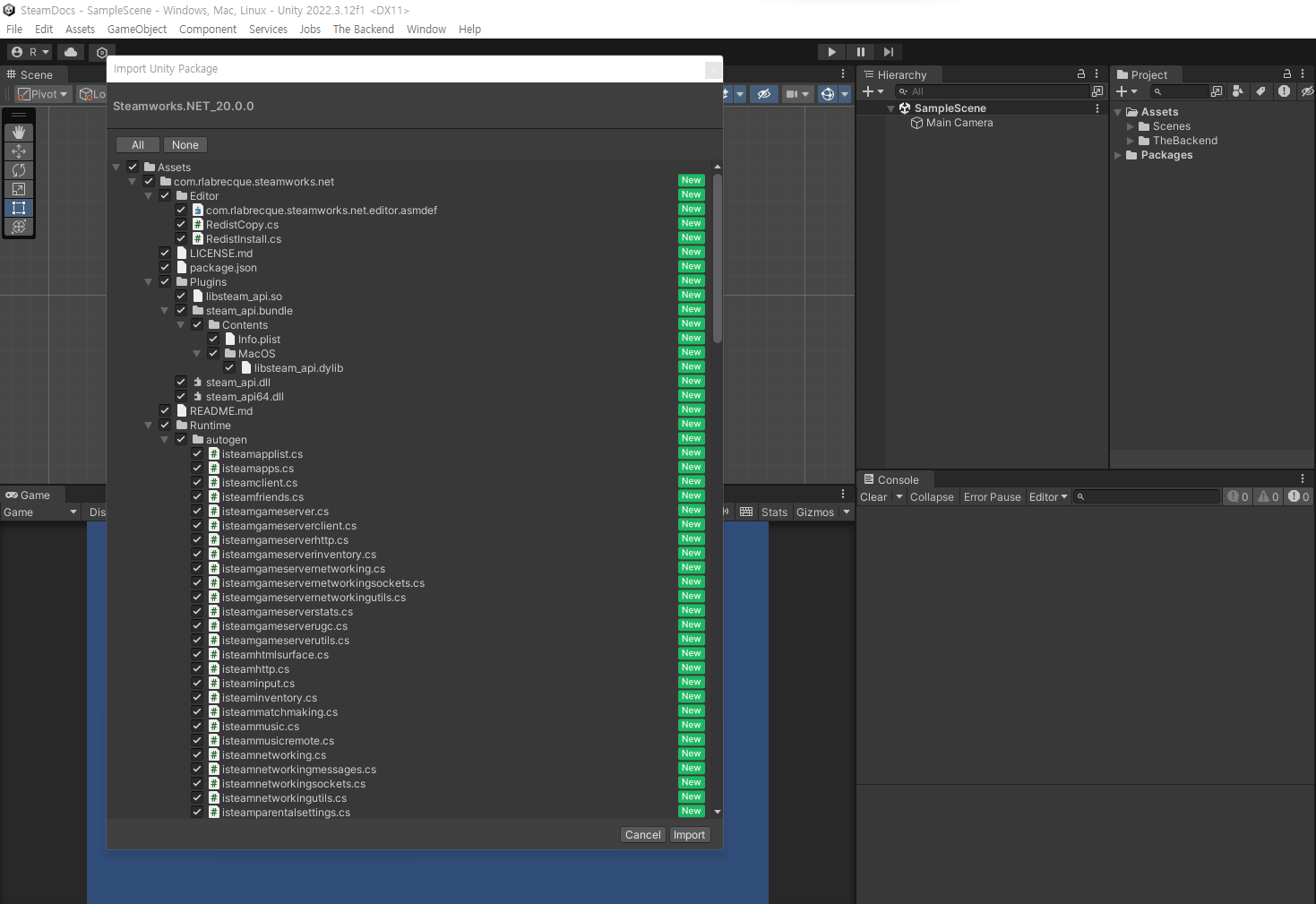
2. Modify steam_appid.txt
Open the steam_appid.txt file in the Unity project folder and enter the Steam appID.
If nothing is entered, 480 will be entered.
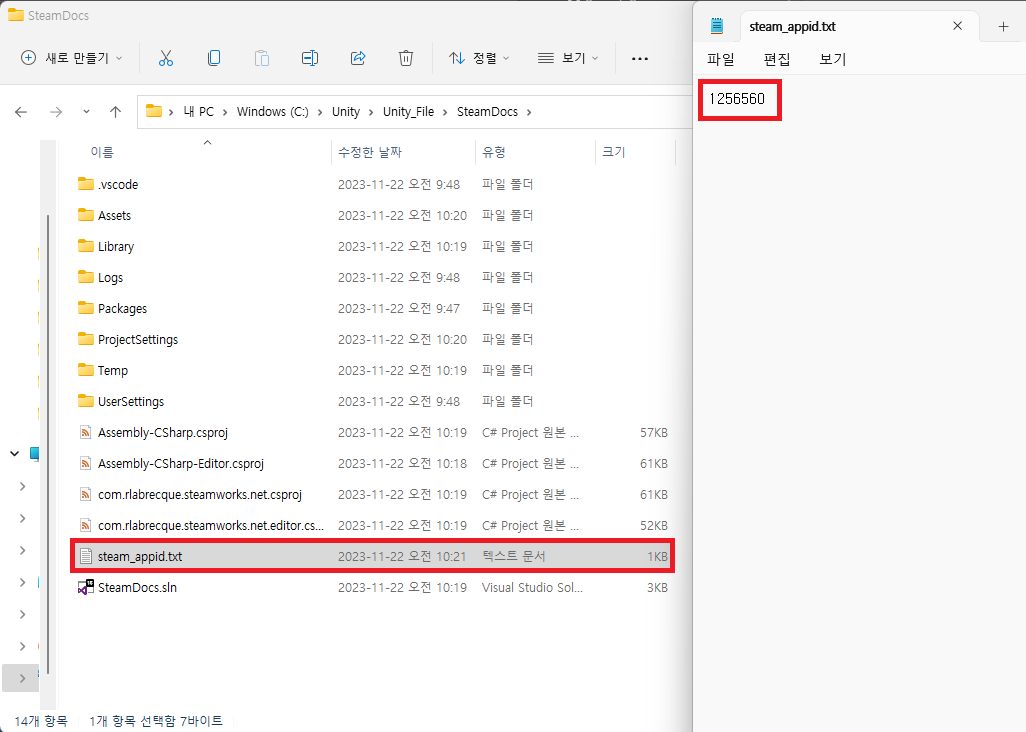
3. Add SteamManager script to a scene
Create an empty object in Hierarchy and add Assets > Scripts > Steamworks.NET > SteamManager.cs as a component.
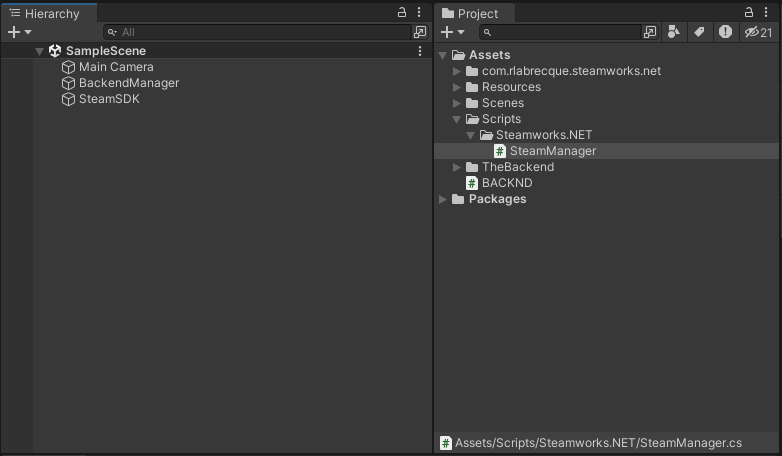
Log in to BACKND server through Steam federation
SocialMediaSignIn
public BackndReturnObject SocialMediaSignIn(string federationToken, FederationType type);
public BackndReturnObject SocialMediaSignIn(string federationToken, FederationType type, string ect);
Parameters
Value | Type | Description |
---|---|---|
federationToken | string | Token value created via each login plugin |
federationType | FederationType | Types of federations (FederationType.Steam) |
ect | string | (Optional) The piece of information desired to be saved from the additional information |
Description
An attempt is made to sign up or log in using Steam's session ticket.
The session ticket value returned from OnGetAuthSessionTicketResponse() can be used to sign up for or log in to the game.
The value of the returned Steam session ticket is as follows:
140000006052bf05a058e52b0d22cb36010010012f435d65180000000100000002000000118c26a42d964b02eec9880001000000c400000044000000040000000d22cb3601001001702c130020c34f341600080a0000000046565c65c60578650100aea806000300626713000000984d29000000a24d290000000000baa2c46a231b8b9ff2def6c5e5e30db4ae8858aaa764a240c8a061203d59ff0f54adaff1920ef8dad9b04aafb04ade640138f3ccf7187e6bf44f79cc60355d5d457ee5a6d1a304dc73ed0861e4a3553b9ad037e17782a1a3474443d18c4db0f830afb18d931a9ba0040e8569b862c63bf81d30854783d77cc828c3d8160ca481
Required namespace
using Steamworks;
Example of a code receiving a Steam session ticket
When the GetAuthSessionTicket method is called from the Start method, the OnGetAuthSessionTicketResponse method will return a value when the call is terminated.
private byte[] m_Ticket;
private uint m_pcbTicket;
private HAuthTicket m_HAuthTicket;
string sessionTicket = string.Empty;
protected Callback<GetAuthSessionTicketResponse_t> m_GetAuthSessionTicketResponse;
void OnGetAuthSessionTicketResponse(GetAuthSessionTicketResponse_t pCallback)
{
//Resize to buffer of 1024
System.Array.Resize(ref m_Ticket, (int)m_pcbTicket);
//format as Hex
System.Text.StringBuilder sb = new System.Text.StringBuilder();
foreach (byte b in m_Ticket) sb.AppendFormat("{0:x2}", b);
sessionTicket = sb.ToString();
Debug.Log("Hex encoded ticket: " + sb.ToString());
}
void Start()
{
Backnd.Initialize();
if (SteamManager.Initialized)
{
m_GetAuthSessionTicketResponse = Callback<GetAuthSessionTicketResponse_t>.Create(OnGetAuthSessionTicketResponse);
m_Ticket = new byte[1024];
m_HAuthTicket = SteamUser.GetAuthSessionTicket(m_Ticket, 1024, out m_pcbTicket);
}
}
Example of a normal code
BackndReturnObject bro = Backnd.Player.SocialMediaSignIn(sessionTicket, FederationType.Steam);
if(bro.IsSuccess())
{
Debug.Log("Login with Steam successful");
//Handled as success
}
else
{
Debug.LogError("Login with Steam failed");
//Handled as failure
}
Other Steam methods
Debug.Log("Steam ID : " + SteamUser.GetSteamID()); // Unique value - number combination
Debug.Log("User nickname : " + SteamFriends.GetPersonaName()); // Nickname - nickname (E.g.: Backnd01 )
Debug.Log("User country information : " + SteamUtils.GetIPCountry()); // User country information - KR
Return cases
Success cases
When the login is successful
statusCode : 200
When signing up as a new member is successful
statusCode : 201
Error cases
When the account is blocked
statusCode : 403
errorCode : Reason for blocking entered in the console