get
BACKND Guidelines > 4. Implementing Chart Functions > Step 3. Loading Chart Information
Loading Chart Information
1. Copy the chart's file ID from BACKND Console
Go to BACKND Console and copy the ID of the chart file uploaded to BACKND Base > Chart Management > Item Chart
.
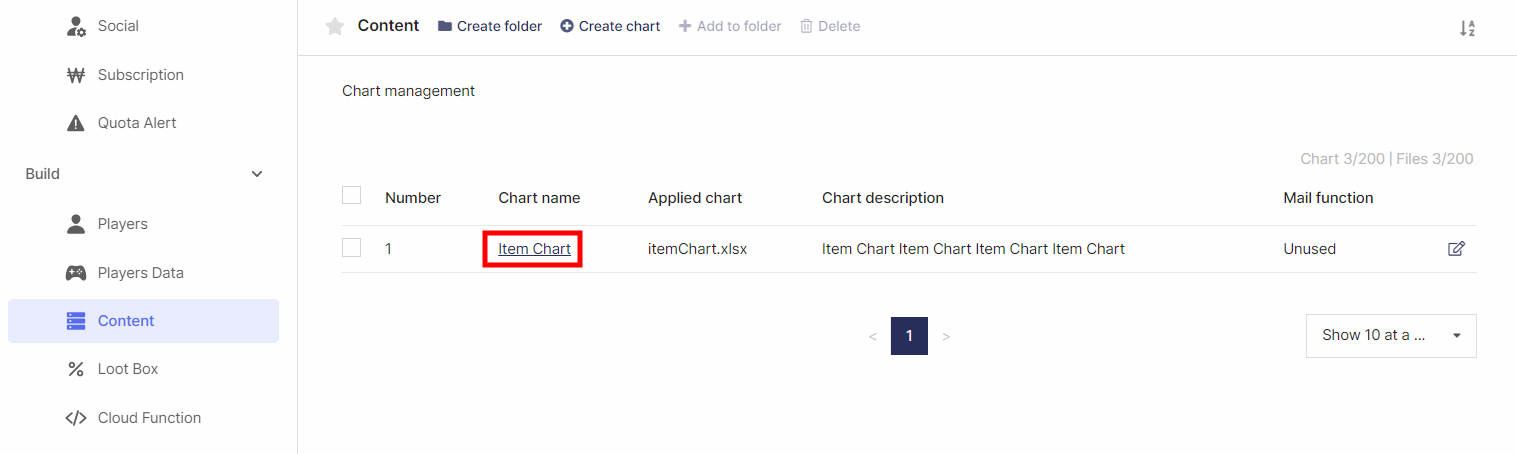
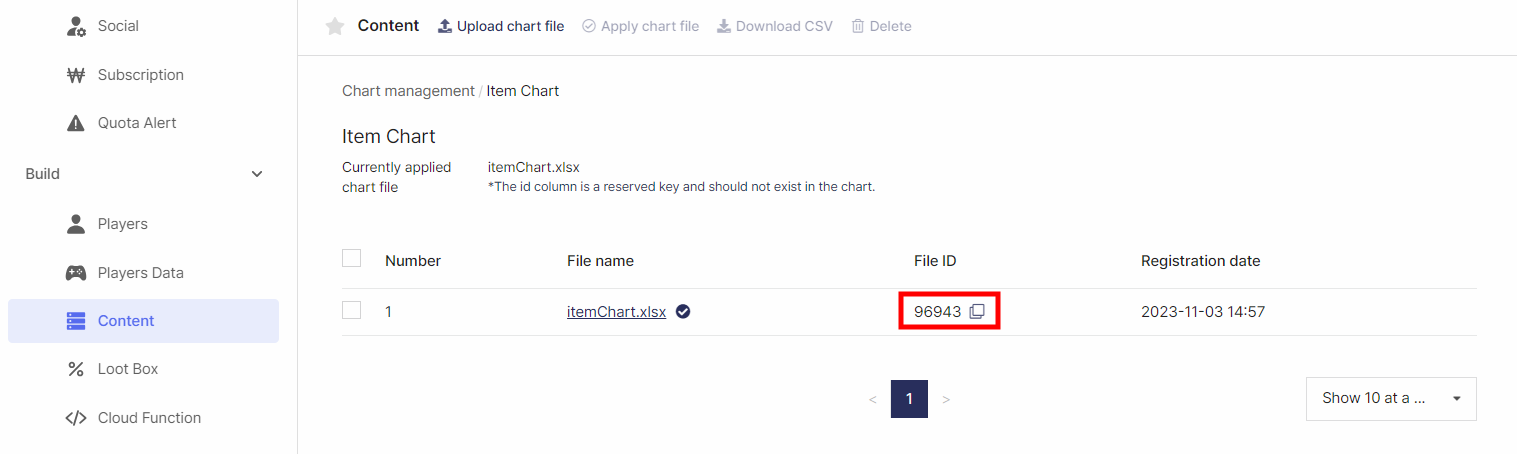
2. Write a chart loading method
Add content to the Chart method in BackendChart.cs written in Pre-arrangements.
BackendChart.cs
Before editing
public void ChartGet(string chartId) {
// Adds content of Step 3. Loading Chart Information
}
After editing
public void ChartGet(string chartId) {
Debug.Log($"Requesting {chartId} chart to be load.");
var bro = Backend.Chart.GetChartContents(chartId);
if(bro.IsSuccess() == false) {
Debug.LogError($"An error occurred while attempting to load {chartId} chart. : " + bro);
return;
}
Debug.Log("Chart loaded successfully. : " + bro);
foreach(LitJson.JsonData gameData in bro.FlattenRows()) {
StringBuilder content = new StringBuilder();
content.AppendLine("itemID : " + int.Parse(gameData["itemId"].ToString()));
content.AppendLine("itemName : " + gameData["itemName"].ToString());
content.AppendLine("itemType : " + gameData["itemType"].ToString());
content.AppendLine("itemID : " + long.Parse(gameData["itemPower"].ToString()));
content.AppendLine("itemInfo : " + gameData["itemInfo"].ToString());
Debug.Log(content.ToString());
}
}
3. Add method call to BackendManager.cs
BackendManager, which is automatically called when the game is executed, must be used to call this method.
Add it so that the method may be called after BACKND initialization and BACKND login.
At this time, paste the chart file ID copied in No. 1 as the parameter value of BackendChart.Instance.ChartGet.
Example: "<File ID>" -> "64584"
BackendManager.cs
Before editing
async void Test() {
await Task.Run(() => {
BackendLogin.Instance.CustomLogin("user1", "1234"); // BACKND login method
// Adds logic for loading chart information
Debug.Log("Test complete.");
});
}
After editing
async void Test() {
await Task.Run(() => {
BackendLogin.Instance.CustomLogin("user1", "1234"); // BACKND login method
// [Addition] Loads chartId's chart information
// [Change required] Change the <File ID> to the file ID value of the chart registered to BACKND Console > Chart Management > Item Chart.
BackendChart.Instance.ChartGet("<File ID>"); // Example: "64584"
Debug.Log("Test complete.");
});
}
4. Test in Unity
After editing the script, execute Unity debugging and check the console log of Unity.
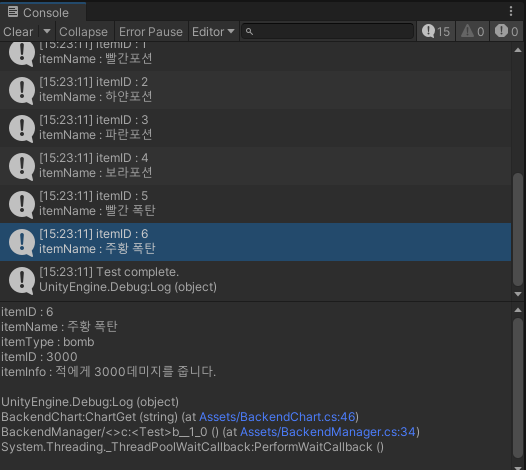
The method has been successfully called when the 'pieces of data registered to the chart' are displayed on the log.
When errors, such as statusCode : 400, 404, and 409, occur instead of the above log, you can check which errors caused the failure in GetChartContents error case.